
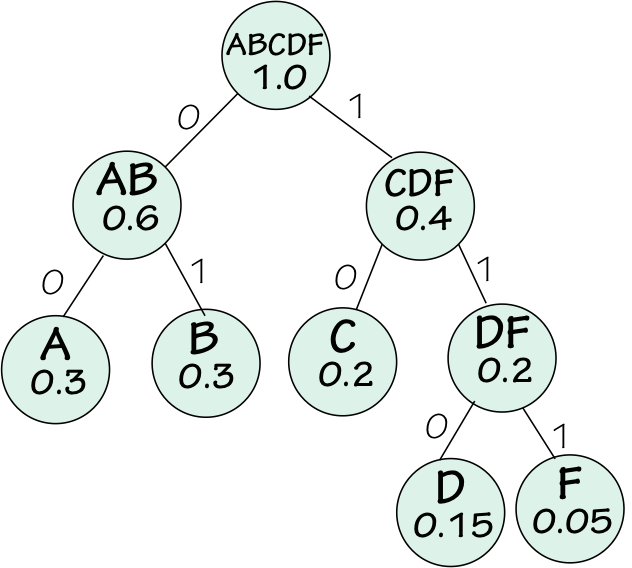
- Huffman treee input vector freq output vector code manual#
- Huffman treee input vector freq output vector code code#
- Huffman treee input vector freq output vector code series#
- Huffman treee input vector freq output vector code windows#
Scripting - Server Side (PHP, Perl, etc.
Huffman treee input vector freq output vector code windows#
Windows Presentation Foundation (WPF) & XAML forum.NET Installation and Configuration Issues Quick Navigation C++ (Non Visual C++ Issues) Top Last edited by Paul McKenzie April 15th, 2010 at 11:42 AM. There are also other places where you use "malloc", when either new/delete or std::vector could be used (the latter being much safer). You shouldn't be using malloc() in a C++ program, unless you're writing your own allocator or using placement-new. The reason is that new calls the object's constructor, and you need a constructor so that the std::string member gets constructed properly. You dynamically create C++objects using "new" not malloc. When your program now attempts to use the std::string member, the std::string was never created.
Huffman treee input vector freq output vector code code#
Right now, your code is using a falsely "created" object, and probably the reason why things go wrong.Īll malloc() does is give you a number of bytes that is equivalent to the sizeof(node)- that's it, nothing else - malloc doesn't create a node object. The node has a std::string member, therefore it must be properly constructed. The node struct is non-POD, therefore you cannot use "malloc" to create such an object. You can't simply copy what 'C' does and use it in C++. If you lifted these lines from a 'C' implementation, then that is your problem. Last edited by monarch_dodra April 15th, 2010 at 06:22 AM.Ĭode: node *min = (node *) malloc(sizeof(*min)) Ĭurr = (node *) malloc(sizeof(*curr)) These lines are totally wrong. Then they think "**** that".ĭid you try running your code through a debugger? This should pinpoint where your code is wrong. HuffmanTree () 2/3 Creates a HuffmanTree from a binary file that has been written to compress the tree information. Parameters frequencies The Frequency objects for this tree. But right now, they just see hundreds of lines of codes, and two classes called "binary tree" and "priority queue". Creates a HuffmanTree from a given set of Frequency objects. I recommend using the above two structures for two reasons:ġ - They are better than what you can write, have proper memory management, less bugsĢ - People here actually want to stop to help. Heck, you never even dealocate! Is this a reason you insist on using things like malloc, calloc realloc, when you are writting c++, and you have the keyword new? It will make your life MUCH easier. On the same note, rather than write your own tree, I recommend you use Is there a reason why you are not using std::priority_queue ( )? I don't know much about huffman, so I can't help you with your algoprithm. Ofstream ofile(argv, ios::out | ios::trunc) Ofstream ofile(hufargv2.c_str(), ios::out | ios::trunc) Int *freqs = (int *) calloc(256, sizeof(*freqs)) Node *min = (node *) malloc(sizeof(*min)) Perhaps use a std::vector for this and convert to string when we need to.While ((v > 1) & (pq.freq > pq.freq))
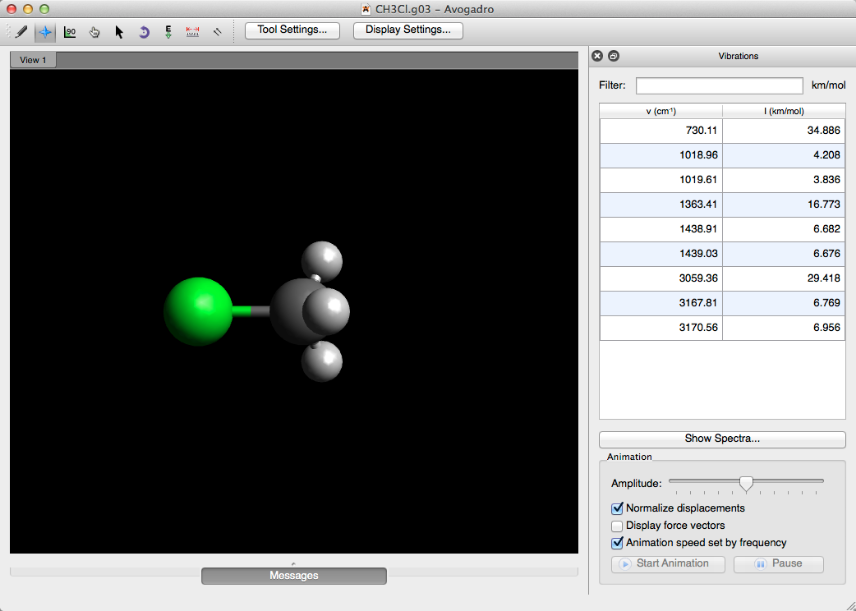
Using a std::string to collect the bits might be quite slow. If (bit_search_traversal(character, node->s_left, bits))
Huffman treee input vector freq output vector code series#
The library consists of two primary functions, huffmanencode () and huffmandecode (), and a series of helper.
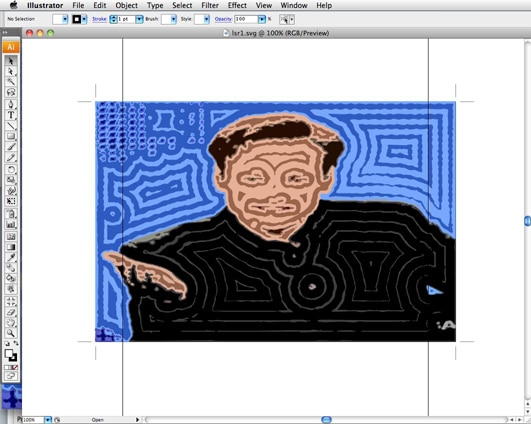
But there's no need to pass a Plain Old Data type like uint8_t by const&. I have written a small library for C that implements the Huffman coding algorithm as outlined in David Huffman's paper on Minimum-Redundancy Codes, and a small test program to implement it. Passing the shared_ptr by const& is good. The argument is already an r-value: min_heap.push(std::make_unique(std::move(node_one), std::move(node_two))) īool HuffmanEncoder::bit_search_traversal(const uint8_t& character, const std::shared_ptr& node, std::string& bits) Node(int16_t frequency, uint64_t c_count) ))) Īgain, we can std::make_shared or std::make_unique, and there's no need for the outermost std::move. I had to work a lot with unique_ptr and shared_ptr in this small assignment, and it's also my first time doing a const_cast which I know should be rarely if at all used.
Huffman treee input vector freq output vector code manual#
So no C-style casting, no manual allocations, and unsafe pointers, etc.
Binary codes are represented by std::vectorDecoding is done by traversing the Huffman tree, as prescribed by the algorithm. This is for a class where we're learning proper modern C++ practices. After the tree is built, a code table that maps a character to a binary code is built from the tree, and used for encoding text. Take any file, read the binary character counts and construct a Huffman tree, and then output the bit code of each character (displayed as its 8-bit value).
